Introduction:
ASP stands for Active server pages.
Released in January 2002.
Web framework developed and designed by Microsoft.
Used to develop web applications and web services.
Provides intergration of html, css and javascript.
Asp.Net is a part of Microsoft.Net framework.
Downloading Visual Studio:
Click on install.
After installing we can see launch click on it.
It will ask sign or click on later.
Click on start visual studio.
After opening click on file-->New-->project.
Left side we can see web option click on it and select Asp.Net web application and click on ok.
Select web forms and click on ok. We can see on right side default.aspx if we click on it we can see default program.
Right click on it and we can see view in browser click on it.
In opera internet browser we can see the output page.
Compilation:
2 types.
1.Explicit compilation:
Converts source code directly to machine code understandable by CPU.
Eg: C, C++
2.Implicit compilation:
Two step process requires virtual machine to execute your code.
Step 1:
Converting program to byte code which is understandable by virtual machine.
.NET bytecode is called Common Intermediate Language or CIL. It is also known as Microsoft Intermediate Language (MSIL) or just Intermediate Language (IL).
Step2:
Converting CIL code to a machine code.
Converts only executed CIL fragments into CPU instructions at runtime.
Just-In-Time Compilation:
Process of converting CIL to machine code translation.
Done by JIT Compiler (JIT or Jitter) which is a part of Common Language Runtime.
Creating new web form:
Open file and then create a project.
Click on that projrct name and add a new item.
Left side we can see web click on it then click on web form and click on add to create a new web form. Default code will be opened write what ever code you required.
I wrote in <h1>tag code and right side we can see webform1.aspx click on it.
After clicking on webform click on view in browser.
Opera browser will be opened we can see the code output in web form.
ASP.Net MVC:
Model View Controller.
Model:
Implements logic for application.
Used to store and retrieve in a database like SQL server.
Uses for business logic.
View:
User interface.
Used to create web pages for application.
View only displays information.
Controller:
Handles user interaction.
Works with model and select view to render web pages.Controller handles and responds to user input and requests.
Differences:
ASP.Net webforms | ASP.Net MVC |
---|---|
Use Page controller pattern. | Uses Front Controller approach. |
No separation. | Very clean separation of concerns. View and Controller are neatly separate. |
Automated testing is really difficult. | Development is quite simple using this approach. |
Follows a Page Life cycle. | No Page Life cycle.Request cycle is simple in ASP.NET MVC model. |
Good for small scale applications with limited team size. | Large-scale applications where different teams are working together. |
Hello world program:
Visual Basic:
Variables:
Variable is a name given to storage area.
Variable initialization:
Initialized with an = sign followed by an constant.
Syntax:
Variable_name = value;
Eg:
Dim pi As double
pi = 3.14
(or)
Dim pi As double = 3.14
Using variables:
In order to use variables first we have to declare.
Variable declaration:
Syntax:
variablename[([boundslist])][As[New]datatype]
Eg:
Dim strName as String //declares a string called strName
DataTypes:
Determines how much space it occupies in storage.
Loops:
Do loop:
Syntax:
Eg:
Control statements:
Continue:
Syntax:
Continue { Do | For |While }
Flowchart:
Goto:
Transfers control to the labelled statement.
Syntax:
GoTo label
Flowchart:
Exit:
Terminates the loop.
Syntax:
Exit { Do | For | Function | Property |select | Sub | Try |While }
Flowchart:
Programs:
Adding two strings:
Partial strings:
Find starting point for a string:
Read from user input and write sentence:
How to write program in notepad and compile in cmd:
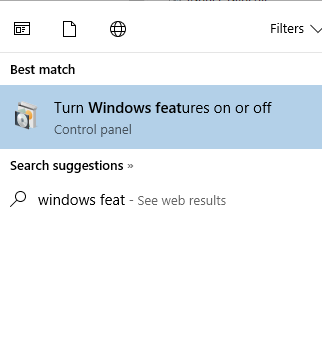
Select .Net Framework and click on ok.
In c drive we can see windows then .Net then framework and select version and copy path and paste in command prompt.
Count the number of occurances of a substring in a string:
Read from a file:
Write to a file:
Read second and fourth line:
Ravi replace with chandu:
First non repeated character:
Visual Basic:
Variables:
Variable is a name given to storage area.
Variable initialization:
Initialized with an = sign followed by an constant.
Syntax:
Variable_name = value;
Eg:
Dim pi As double
pi = 3.14
(or)
Dim pi As double = 3.14
Using variables:
In order to use variables first we have to declare.
Variable declaration:
Syntax:
variablename[([boundslist])][As[New]datatype]
Eg:
Dim strName as String //declares a string called strName
DataTypes:
Determines how much space it occupies in storage.
Data Type | Storage Allocation | Value Range |
---|---|---|
Boolean | Depends on implementing platform | True or False |
Byte | 1 byte | 0 through 255 (unsigned) |
Char | 2 bytes | 0 through 65535 (unsigned) |
Date | 8 bytes | 0:00:00 (midnight) on January 1, 0001 through 11:59:59 PM on December 31, 9999 |
Decimal | 16 bytes | 0 through +/-79,228,162,514,264,337,593,543,950,335 (+/-7.9...E+28) with no decimal point; 0 through +/-7.9228162514264337593543950335 with 28 places to the right of the decimal |
Double | 8 bytes |
-1.79769313486231570E+308 through -4.94065645841246544E-324, for negative values
4.94065645841246544E-324 through 1.79769313486231570E+308, for positive values
|
Integer | 4 bytes | -2,147,483,648 through 2,147,483,647 (signed) |
Long | 8 bytes | -9,223,372,036,854,775,808 through 9,223,372,036,854,775,807(signed) |
Object |
4 bytes on 32-bit platform
8 bytes on 64-bit platform
| Any type can be stored in a variable of type Object |
SByte | 1 byte | -128 through 127 (signed) |
Short | 2 bytes | -32,768 through 32,767 (signed) |
Single | 4 bytes |
-3.4028235E+38 through -1.401298E-45 for negative values;
1.401298E-45 through 3.4028235E+38 for positive values
|
String | Depends on implementing platform | 0 to approximately 2 billion Unicode characters |
UInteger | 4 bytes | 0 through 4,294,967,295 (unsigned) |
ULong | 8 bytes | 0 through 18,446,744,073,709,551,615 (unsigned) |
User-Defined | Depends on implementing platform | Each member of the structure has a range determined by its data type and independent of the ranges of the other members |
UShort | 2 bytes | 0 through 65,535 (unsigned) |
Do loop:
Syntax:
Do { While | Until } condition
[ statements ]
[ Continue Do ]
[ statements ]
[ Exit Do ]
[ statements ]
Loop
-or-
Do
[ statements ]
[ Continue Do ]
[ statements ]
[ Exit Do ]
[ statements ]
Loop { While | Until } condition
Flow chart:Eg:
For..Next loop:
Repeats a group of statements specific number of times.
Syntax:
For counter [ As datatype ] = start To end [ Step step ]
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ counter ]
Flowchart:
Eg:
For each next:
Repeats group of statements for each element in a collection.
Syntax:
For Each element [ As datatype ] In group
[ statements ]
[ Continue For ]
[ statements ]
[ Exit For ]
[ statements ]
Next [ element ]
Eg:
While...Endwhile loop:
Executes a series of statements as long as given condition is true.
Syntax:
While condition
[ statements ]
[ Continue While ]
[ statements ]
[ Exit While ]
[ statements ]
End While
Flowchart:
Eg:Control statements:
Continue:
Syntax:
Continue { Do | For |While }
Flowchart:
Goto:
Transfers control to the labelled statement.
Syntax:
GoTo label
Flowchart:
Exit:
Terminates the loop.
Syntax:
Exit { Do | For | Function | Property |select | Sub | Try |While }
Flowchart:
Programs:
Adding two strings:
Partial strings:
Find starting point for a string:
Read from user input and write sentence:
How to write program in notepad and compile in cmd:
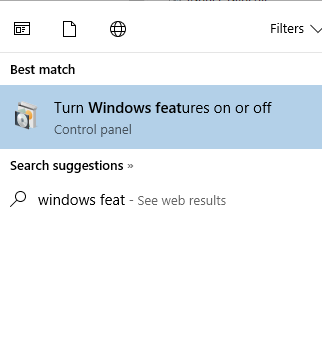
Select .Net Framework and click on ok.
In c drive we can see windows then .Net then framework and select version and copy path and paste in command prompt.
Count the number of occurances of a substring in a string:
Read from a file:
Write to a file:
Read second and fourth line:
Ravi replace with chandu:
First non repeated character:
Permutation of a string: