What is a C Language:
It is a programming language developed by Dennis Ritche at Bell laboratories.
History of C:
It is developed to over come the features of previous languages such as B, BCPL.
Downloading c compiler:
Open google and download turboc for windows.
We can see the download option then click on it.
We can see the zip file has been downloaded.After downloading right click on it and click on show in folder.
After that file will be shown in our dive the right click on the file and click on extract all.
It will ask where the file should be extracted browse where you want to store the file and click on extract.We can see Turco C++ 3.2 has been extracted.
Installing:
Click on the Turbo C++ file and click on next to install.
Click on the radio button and click next.Click on install.
We can see Turbo C is installing.
Files are successfully copied then click on finish.
Now in start enter turbo C++ we can see it has been successfully installed and ready to open.
Click on it and click start Turbo C++.
We can see a blue colour screen has been opened successfully.
Hello C program:
#include <stdio.h> ---- standard input output library functions.
#include <conio.h> ---- console input output library functions.
void main() ---- main() function is the entry point of every program.
void means it return no value.
printf() ---- used to print data on console.
getch() ---- asks for a single character. press any key.
Variable:
Name of memory location.
Used to store data.
Syntax:
type variable_list;
Eg:
Syntax for Variable initiation:
data_type variable_name = constant / literal / expression;
(or)
variable_name = constant / literal / expression;
Eg:
int a = 10;
Types of variables:
Local variable:
Declared inside the function or block.
Eg:
void function() {
int x = 10; // local variable
}
Global variable:
Declared outside the function or block.
Available to all functions.
Eg:
int value = 20; // global variable
void function() {
int x = 10; // local variable
}
Static variable:
Declared with static keyword.
Eg:
void function() {
int x = 10; // local variable
static int y = 10; // static variable
x = x + 1;
y = y + 1;
printf("%d %d",x,y);
}
Automatic variable:
All variables in c that is declared inside the block are automatic by default.
Eg:
void main() {
int x = 10; // local variable (also automatic)
auto int y = 20; //automatic variable
}
External variable:
To declare external variable we have to use extern keyword.
Eg:
myfile.h
extern int x = 10; //external variable (also global)
program1.c
#include "myfile.h"
#include <stdio.h>
void printValue() {
printf("Global variable: %d", global_variable);
}
Data Types:
Data Types | Memory Size | Range |
---|---|---|
char | 1 byte | −128 to 127 |
signed char | 1 byte | −128 to 127 |
unsigned char | 1 byte | 0 to 255 |
short | 2 byte | −32,768 to 32,767 |
signed short | 2 byte | −32,768 to 32,767 |
unsigned short | 2 byte | 0 to 65,535 |
int | 2 byte | −32,768 to 32,767 |
signed int | 2 byte | −32,768 to 32,767 |
unsigned int | 2 byte | 0 to 65,535 |
short int | 2 byte | −32,768 to 32,767 |
signed short int | 2 byte | −32,768 to 32,767 |
unsigned short int | 2 byte | 0 to 65,535 |
long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
signed long int | 4 byte | -2,147,483,648 to 2,147,483,647 |
unsigned long int | 4 byte | 0 to 4,294,967,295 |
float | 4 byte | |
double | 8 byte | |
long double | 10 byte |
Iterate a code or group of code many times.
Advantage:
Saves code.
Types:
for:
Iterates the code until condition is false.
Number of iterations is known by the user.
Syntax:
for(initialization; condition; increment / decrement) {
//code to be executed
}
Flowchart:
while:
While loop statement is given before the statement.
Execute statements 0 or many times.
Syntax:
while(condition) {
//code to be executed
}
Flow chart:
Do-while:
Statement is given before the condition.
Code will be executed at least once.
Syntax:
do {
//code to be executed
}while(condition);
Flow chart:
Control statements:
if statement:
Code executed if condition is true.
Syntax:
if(expression) {
//code to be executed
}
Flow chart:
if else statement:
Executes the code if condition is true or false.
Syntax:
if(expression) {
//code to be executed if condition is true
} else {
//code to be executed if condition is false
}
Flow chart:
if else if ladder:
Executes one code from multiple conditions.
Syntax:
if(condition1) {
//code to be executed if condition1 is true
} else if(condition2) {
//code to be executed if condition2 is true
}
else if(condition3) {
//code to be executed if condition3 is true
}
...
else {
//code to be executed if all conditions are false
}
Flow chart:
Switch:
Used to execute the code from multiple conditions.
Syntax:
switch(expression) {
case value1:
//code to be executed;
break;
case value2:
//code to be executed;
break;
....
default:
//code to be executed if all cases are not matched;
}
Flow chart:
Break:
Break the execution of loop.
Syntax:
jump-statement;
break;
Flowchart:
Continue:
Continue the execution of loop.
Syntax:
jump-statement;
continue;
Goto:
Unconditionally jump to other label.
Transfers control to other parts of the program.
Syntax:
go-to label;
String representation:
Syntax for declaring:
char String_Variable_name [SIZE];
Eg:
char city [30];
char name [20];
Initializing:
3 ways.
char name [] = {'H' , 'E' , 'L' , 'L' , 'O' , '\0'};
char name [ ] = "HELLO";
char *name = "HELLO";
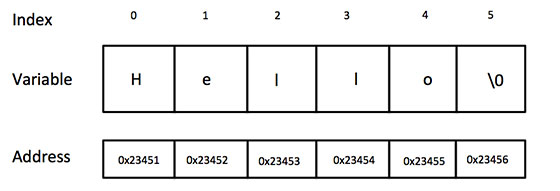
Add two strings:
Partial string:
Read from user input and write to user output:
Graphic output syntax:
Line:
Circle:
Rectangle:
Star:
Reading and writing a file:
Reading a file:
Create a .txt file in notepad and save with .txt file extension.
Copy the created text file in turboc3.
Writing a file:
Open c drive.Open turboc3.
In that we can see bin folder.
Now, we created in our program mouni folder now automatically it creates folder with that name.
After opening that folder we can see data which we have written in our file.
First non repeated character:
Permutation of a string:
Digit identification:
Replacing first highest repeated character:
Pattern:
Sum a number in a given string: